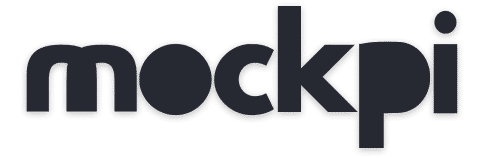
Getting Started>
Quick setup>
Creating & using a pi>
Create a simple pi>
Understanding the components>
Create a dynamic pi>
Pi Configuration>
Components of a pi>
Response configuration>
Dynamic data & faker>
State management>
Webhook configuration>
API References>
Create a new pi>
Find one pi by id>
Get all pies>
Update by id>
Delete a pi>
Getting Started
Welcome to the mockpi documentation!
Here, you’ll find everything you need to get started with mockpi, from creating your first pi (mock API) to advanced configurations.
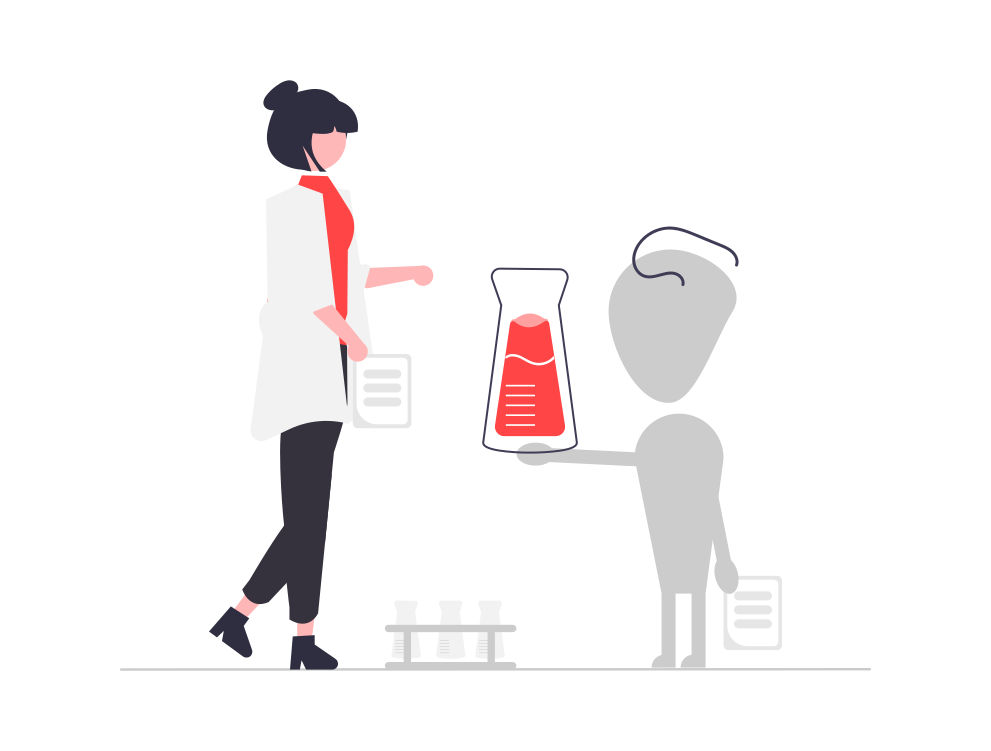
Quick setup
Mockpi is ready to use right out of the box with no additional setup, code changes, or integrations required! Whether you need to mock APIs for local development, UAT, or automation suites, mockpi is versatile and covers all scenarios seamlessly.
Before you get started, please make sure you have access to -
At mockpi, we refer to a mock API as a “pi” (pie). So whenever you see the term "pi", it means the mock API created to simulate your actual API.
Before you get started, please make sure you have access to -
- A free trial or paid subscription (visit mockpi.co/pricing to sign up). That's it!
- Visit API Studio to create API mocks using our powerful piEditor.
- And, If you'd like to use our APIs to create mocks with your own tools, such as Postman, Hopscotch, or custom scripts:
- An API key, which you can retrieve directly from the mockpi dashboard.
- Your Mockpi domain, required to integrate and use the mocks you create. No API Key required for this!
At mockpi, we refer to a mock API as a “pi” (pie). So whenever you see the term "pi", it means the mock API created to simulate your actual API.
Creating & using a pi
Mockpi is a cloud-based API mocking framework that allows you to create and manage mocks without modifying your code. It provides a structure for handling your “pies” (mock APIs) independently, enabling you to use them just like your actual APIs.
Creating and using a “pi” is pretty simple-
So let's create a pi and see how it works!
Creating and using a “pi” is pretty simple-
- Create a pi using our piEditor OR our APIs (see API documentation below).
- Use the pi in your code by simply updating the base URL in your environment variable or configuration settings.
So let's create a pi and see how it works!
Create a simple pi
Let's create a simple mock for Zendesk's Create Ticket API that accepts ticket details and returns a positive response confirming the ticket has been created.
And that’s it! Your pi is created and ready to use, allowing you to simulate the actual API.
Actual API endpoint that you want to mock - https://{subdomain}.zendesk.com/api/v2/tickets.json
And you expect the below JSON body in response with HTTP code as 201
{
"ticket": {
"assignee_id": 235323,
"created_at": "2009-07-20T22:55:29Z",
"priority": "urgent",
"subject": "My printer is on fire!",
"description": "The fire is very colorful."
}
}
Here is what you have to do in order to create a pi (mock API) for that -
Step 1 - Use the below curl request to create the pi ( This curl creates a pi for you. Replace
api-key
header with your API Key ). You do not need any api key if you are using our piEditorcurl --location 'https://api.mockpi.co/v2/api/admin/pi' \
--header 'api-key: your_api_key' \
--header 'Content-Type: application/json' \
--data '{
"method": "POST",
"path": "/api/v2/tickets.json",
"response": {
"code": 201,
"body": {
"ticket": {
"assignee_id": 235323,
"created_at": "2009-07-20T22:55:29Z",
"priority": "urgent",
"subject": "My printer is on fire!",
"description": "The fire is very colorful."
}
}
}
}'
And that’s it! Your pi is created and ready to use, allowing you to simulate the actual API.
Step 2 - Replace Zendesk's base URL with your mockpi domain, which will be in the format
your_unique_domain_name.mockpi.co
You can find this domain in your mockpi dashboard. You can call this API using your_unique_domain_name.mockpi.cc/api/v2/tickets.json
And get the result you just configured.And if you notice, the only difference between the actual api & the pi is the base url. Let's break down each component in the next section.
Understanding above pi & it’s components
- Request payload -
method
- HTTP method of the actual API that you want to mock.path
- Path of the actual API that you want to mock. This starts with a/
and does not include the base URL.response
- You configure the desired response (behaviour & body) here.response.code
- The desired HTTP response code from the piresponse.body
- The desired JSON body in the response code of the pi- Admin API URL -
https://api.mockpi.co/v2/api/admin/pi
(if using API's) - This is the admin pi management endpoint that you use to create & manage pies. You can read API references below to understand all the use cases.
- Header -
api-key
(if using API's) - This is the header where you send your API Key to access the pi management APIs. You can obtain the API key by logging in at mockpi.co.
Create a dynamic pi
The pi we just created returns the same static response every time, which may not be very intuitive. Let’s make it dynamic.
Let's change -
Let's change -
assignee_id
- to return a random id every timecreated_at
- to return the current timestamp every time in UTC formatpriority
- to returnurgent
70% if the times,medium
20% of the times,normal
10% of the times.
curl --location --request PUT 'https://api.mockpi.co/v2/api/admin/pi/{id_of_the_created_pi} \
--header 'api-key: your_api_key \
--header 'Content-Type: application/json' \
--data '{
"method": "POST",
"path": "/api/v2/tickets.json",
"response": {
"code": 201,
"body": {
"ticket": {
"assignee_id": {
"type": "Faker.number.int"
},
"description": "The smoke is very colorful.",
"priority": {
"type": "Faker.string.enum",
"enum": ["urgent", "medium", "normal"],
"enumWeight": [70, 20, 10]
},
"subject": "My printer is on fire!",
"created_at": {
"type": "Faker.date.now"
}
}
}
}
}'
You get the id of the pi in the response when you create it. Or you can use the GET all pies API from the API references. For now if you do not remember the id, you can just create a new API by changing the path a little bit.
Now, if you call the above pi you just created you would a different response every time with dynamic values in
assignee_id, created_at, priority
fields.Let's understand what we just did -
{ "type": "Faker.number.int" }
- This is a dynamic type field configuration. This generates a random int value everytime for the field it is assigned to. So whenever this pi is called, a random value is generated for assignee_id field because of this type configuration.- This is a way to generate random data using a faker library. You can do much more with dynamic type field which is explained in the section below.
{ "type": "Faker.date.now" }
- Similarly, this generates the current timestamp for created_at field whenever this pi is called.{ "type": "Faker.string.enum", "enum": ["urgent", "medium", "normal"], "enumWeight": [70, 20, 10] }
- This dynamic type configuration picks a random item from the list of enum provided and populates into the given field.
- As we have also configured enumWeight, instead of picking an item randomly - it picks it based on its weight. So if you call this pi 100 times, almost 70 times, you would get the value of priority as urgent.
- The weight calculation is performed probabilistically, meaning it doesn't guarantee that you'll receive the item exactly 70% of the time.
You can refer to the next section to understand all the features & it's usage.
If you face any issues - Feel free to reach out to us at support@mockpi.co for more help!
Pi Configuration
Pi is supported by a robust configuration language that provides the flexibility to create complex mock APIs with ease. It’s simple to configure yet powerful enough to enable intricate API behaviors beyond just dynamic data such as -
- Generating realistic random data
- Embedding request input data into response bodies
- Performing request matching for conditional responses
- Managing states between APIs
- Forwarding requests or calling webhooks
- Responding with dynamic & weight based HTTP codes
- Mimicking custom latencies or timeouts
- Formatting response with prefixes, date formats, casing and much more
Components of a pi
When creating a pi, you have access to a powerful suite of configuration options that allow you to design complex API responses and behaviors. Let’s dive into each of these components in detail -
Field | Data type | Description / Usage |
---|---|---|
method | String | Required This specifies the HTTP method of the actual API you wish to mock. Ex - GET , POST , etc. See all the allowed methods here - https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods |
path | String | Required This represents the endpoint of the actual API you want to mock, excluding the base URL or host, and it begins with a slash (/ ).For example, to mock https://zendesk.com/api/ticket/details , the path would be /api/ticket/details You can also include dynamic parameters in the path, such as /api/ticket/details/:ticketId , which matches any API paths that start with /api/ticket/details/ followed by a dynamic value. This would match paths like /api/ticket/details/12345 , /api/ticket/details/abcd123456 and so on. |
project | String | Optional Defaults to none This field identifies the project under which you’re creating the API mock. Typically, it’s not necessary to use this unless you have a scenario where multiple teams in your company need to mock the same API in total separation. You can leave it empty if not needed. If selected, all mock APIs under this project will be prefixed with the project name. |
response | JSON ({} or [{}]) | Optional This defines the expected response from the pi, including settings like HTTP status codes, latency, response body, weight and pattern-based responses, request-to-response data forwarding, webhooks trigger, and more. You can configure one response or multiple, by using JSON array. For a detailed breakdown, refer to the Response section. |
state | JSON ({}) | Optional This configuration allows state management across APIs, enabling you to use incoming data from one API in the response of another. See the State section for more details. |
Let's take a look at couple of samples -
/** Example 1 */
{
"method": "GET",
/** Match dynamic ticketId */
"path": "/api/v2/tickets/:ticketId",
/** Single response */
"response": {
"code": 201,
"body": {
"ticket": {
/** Dynamic number */
"assignee_id": {
"type": "Faker.number.int"
},
"priority": "urgent",
/** Populate subject from the incoming request */
"subject": {
"type": "Request.body.subject"
},
"description": "The fire is very colorful.",
/** Dynamic current timestamp */
"created_at": {
"type": "Faker.date.now"
}
}
}
}
}
/** Example 1 */
{
"method": "GET",
/** Match dynamic ticketId */
"path": "/api/v2/tickets/:ticketId",
/** Multiple response configuration */
"response": [{
/** Response 1 with code 201 - approx 70% of the times */
"code": 201,
"weight": 70,
"body": {
"ticket": {
/** Dynamic number */
"assignee_id": {
"type": "Faker.number.int"
},
"priority": "urgent",
/** Populate subject from the incoming request */
"subject": {
"type": "Request.body.subject"
},
"description": "The fire is very colorful.",
/** Dynamic current timestamp */
"created_at": {
"type": "Faker.date.now"
}
}
}
}, {
/** Response 2 with code 400 - approx 30% of the times */
"code": 400,
"weight": 30,
"body": {
"error": "ticket not found!",
"ticket": null
}
}]
}
Response configuration
You can configure one or more desired responses for the pi using either a single JSON object or an array of JSON objects. Each JSON object can include the following fields:
Field | Data type | Description / Usage |
---|---|---|
code | String | Optional Defaults to 200 This is the desired response HTTP code. Ex - 200 , 400 , etc. |
body | JSON ({}) | Optional Defaults to {} This is the desired response body configuration. Read the next section for response body configuration options. |
latency | Number or Number[] | Optional This is the list of latency you want for the pi response. You can specify one or more values, and if multiple values are provided, a random latency will be selected unless a latencyWeight is specified. |
latencyWeight | Number[] | Optional Weight of the latency mentioned. If provided, the latency is calculated based on weight. |
match | JSON or JSON[] | Optional Match conditions to determine whether this response should be selected. If the match is successful, the corresponding response will be chosen. This condition has the highest priority in relation to weighted and random responses. |
contentType | String | Optional Defaults to application/json You can also configure static HTML (text/html ) & XML (application/xml ) using this. |
webhook | JSON | Optional You can configure a webhook for each response that gets triggered after the response is sent. You can create dynamic webhooks with delay as well. |
Dynamic response body & faker
The dynamic response data of the pi is driven by a custom field type that offers various options for generating data. You can add prefixes, apply formatting options, utilize the Faker.js library for data generation, incorporate incoming request data into the response, and use state data as well.
To fill any field with dynamic data, simply replace the value using the configurations object below:
Field | Data type | Description / Usage |
---|---|---|
type | String | Optional This has 3 types - Faker type like Faker.string.enum , Request type Request.body.anyKeyName & State type State.body.anyKeyName If the type provided matches the configuration then a dynamic data is populated otherwise it is considered as a nested JSON and returned as it is. |
prefix | String | Optional If provided, this string as added as a prefix to the generated value by the type. |
suffix | String | Optional If provided, this string as added as a suffix to the generated value by the type. |
lowercase | Boolean (true) | Optional If provided as true, the whole value will be changed to lowercase. |
uppercase | Boolean (true) | Optional If provided as true, the whole value will be changed to uppercase. |
max | Number | Optional if provided, only a substring of the value is populated. |
format | String | Optional Applicable for Date data types only. If provided, the date is converted into given format i.e. YYYY-MM-DD, DD-MM-YYYY, MM-DD-YYYY, YYYY/MM/DD etc |
enum | String[] or Number[] | Optional Applicable for Faker.number.enum & Faker.string.enum if provided, a random value from the given enum is picked. |
weight | Number[] | Optional Applicable for above enum types. if provided, a value from the given enum is picked based on respective weight. |
options | JSON | Optional Options for faker.js api function. See here - https://fakerjs.dev/api/person.html#fullname. |
Here are some dynamic body samples:
{
/** Generate a random name using faker */
"name": {
"type": "Faker.person.name"
},
/** Static value as it is */
"bio": "What a day! Enjoy.",
/** Generate value with prefix and uppercase */
"handle": {
"type": "Faker.string.numeric",
"prefix": "ID_",
"uppercase": true
},
/** Populate id from the incoming request body */
"id": {
"type": "Request.body.userId"
},
/** Populate email of the user that was stored from another api */
"email": {
"type": "State.body.email"
},
/** Nested JSON as it is as this does not match a dynamic type */
"address": {
/** Static value as it is */
"street": "47 W 13th St",
"city": "New York",
"country": "USA",
/** Populate dynamic phone value from faker */
"phone": {
"type": "Faker.phone.number",
/** faker.js options */
"options": { "style": "international" }
}
},
/** This JSON would be reurned as it as the type is not a dynamic type */
"description": {
"type": "Individual",
"organization": "Self owned"
},
/** You can have any nested levels */
"details": {
"field1": "value1",
"field2": {
"names": ["Name 1", "Name 2"],
"field3": {
"data": "inside data"
}
}
}
}
State management
State management allows you to configure APIs to maintain context between one another, enabling you to use the request data from one API in the responses of another. Below are the configuration options:
Field | Data type | Description / Usage |
---|---|---|
id | String | Required This is the identifier field against which a state is managed. Values could be Request.body.anyKeyName, Request.params.anyKeyName , etc. |
headers | String[] | Optional List of API headers to store in the state like [Request.headers.fieldName] |
body | String[] | Optional List of body fields to store in the state like [Request.body.fieldName, Request.body.field2.field12] |
params | String[] | Optional List of API param fields to store in the state like [Request.params.fieldName] |
queries | String[] | Optional List of API query fields to store in the state like [Request.queries.fieldName] |
Webhook configuration
You can configure a webhook for each response that gets triggered after the response is sent. Below are the configuration options:
Field | Data type | Description / Usage |
---|---|---|
delay | Number (seconds) | Optional Webhook is triggered after a delay of given seconds. Max allowed value is 60 (1 minute). |
method | String | Optional Defaults to GET The HTTP method of the webhook API. |
url | String | Required Fully qualified webhook URL |
headers | JSON | Optional Headers to be included in the webhook API like {Authorization: "some_api_key"} |
body | JSON | Optional Webhook request body like {name: "Joseph", gender: "male"} |
If you face any issues - Feel free to reach out to us at support@mockpi.co for more help!
API References for pies
Mockpi offers a set of APIs that you can use to manage all your pies (mock APIs). These APIs provide the flexibility to integrate with any tool you choose and create configurations of any complexity. They are secured and can only be accessed using your admin API keys, which you can create and manage from the team section in the Mockpi dashboard.
Find below details to use these API:
Field | Value | Description / Usage |
---|---|---|
Base URL | https://api.mockpi.co | This is the mockpi base URL for managing the APIs. Note that this is different from the mockpi domain that you get to use your pies. |
Header | api-key | Each API requires this header in order to authenticate and authorise you. |
Header | Content-Type | All the APIs are application/json |
Current version | v2 | This is the current version of pi management APIs. |
Create a new pi
API -
POST
/v2/api/admin/pi
This API is used to create a new pi. A pi is unique for a given method and path so two pi cannot be created with same values. The api returns a response with details like pi id, sample pi endpoint to use it etc if successful otherwise an error message is returned.
Request Payload -
Field | Data type | Description / Usage |
---|---|---|
method | String | Required This specifies the HTTP method of the actual API you wish to mock. Ex - GET , POST , etc. See all the allowed methods here - https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods |
path | String | Required This represents the endpoint of the actual API you want to mock, excluding the base URL or host, and it begins with a slash (/ ).For example, to mock https://zendesk.com/api/ticket/details , the path would be /api/ticket/details You can also include dynamic parameters in the path, such as /api/ticket/details/:ticketId , which matches any API paths that start with /api/ticket/details/ followed by a dynamic value. This would match paths like /api/ticket/details/12345 , /api/ticket/details/abcd123456 and so on. |
project | String | Optional Defaults to none This field identifies the project under which you’re creating the API mock. Typically, it’s not necessary to use this unless you have a scenario where multiple teams in your company need to mock the same API in total separation. You can leave it empty if not needed. If selected, all mock APIs under this project will be prefixed with the project name. |
response | JSON ({} or [{}]) | Optional This defines the expected response from the pi, including settings like HTTP status codes, latency, response body, weight and pattern-based responses, request-to-response data forwarding, webhooks trigger, and more. You can configure one response or multiple, by using JSON array. For a detailed breakdown, refer to the Response section. |
state | JSON ({}) | Optional This configuration allows state management across APIs, enabling you to use incoming data from one API in the response of another. See the State section for more details. |
Response data -
Field | Data type | Description / Usage |
---|---|---|
success | Boolean | true if the pi is created successfully |
data.id | String | Id of the creayed pi that you can use in further APIs |
data.piUrl | String | A sample URL to use you pi. This would be of the pattern - your_company.mockpi.co/your_api_path |
error | String | Error message if something went wrong while creating the pi |
See below sample request -
curl --location 'https://api.mockpi.co/v2/api/admin/pi' \
--header 'api-key: your_api_key' \
--header 'Content-Type: application/json' \
--data '{
"method": "GET",
"path": "/api/v2/tickets/:ticketId",
"response": {
"code": 201,
"body": {
"ticket": {
"assignee_id": {
"type": "Faker.number.int"
},
"priority": "urgent",
"subject": {
"type": "Request.body.subject"
},
"description": "The fire is very colorful.",
"created_at": {
"type": "Faker.date.now"
}
}
}
}
}'
A successful response of above request would look like this -
{
"success": true,
"data": {
"id": "671aa75643075f8841becb27",
"piUrl": "https://your_company.mockpi.co/api/v2/tickets/:ticketId"
}
}
Find pi by id
API -
GET
/v2/api/admin/pi/:id
This API is used to get an already created pi.
See below sample request -
curl --location 'https://api.mockpi.co/v2/api/admin/pi/670ad5d21051b93f61ffd64f' \
--header 'api-key: your_api_key'
The response of this API is the pi you created -
{
"success": true,
"data": {
"id": "671aa68acdeb237789b2bfa2",
"method": "GET",
"path": "/zendesk/user/:userId",
"response": {
"code": 200,
"body": {
"firstName": "Joey",
"lastName": {
"type": "Faker.person.lastName",
"length": 5
},
"gender": {
"type": "Faker.string.enum",
"enum": [
"Male",
"Female"
]
}
},
"contentType": "application/json"
}
}
}
Get all pies
API -
GET
/v2/api/admin/pi
This API is used to get all pies that you have created till now.
See below sample request -
curl --location 'https://api.mockpi.co/v2/api/admin/pi' \
--header 'api-key: your_api_key'
See below sample that has multiple pies in the response -
{
"success": true,
"data": [
{
"id": "671aa68acdeb237789b2bfa2",
"method": "GET",
"path": "/user/details",
"response": {
/** response configuration of this api */
}
},
{
"id": "671aa75643075f8841becb27",
"method": "GET",
"path": "/user/details",
"response": [
/** response configuration of this api */
]
}
]
}
Update a pi by id
API -
PUT
/v2/api/admin/pi/:id
This API is used to update an existing pi. The request payload and response of this API is exactly same as the Create pi API.
Delete pi by id
API -
DELETE
/v2/api/admin/pi/:id
This API is used to delete an already created pi.
See below sample request -
curl --location --request DELETE 'https://api.mockpi.co/v2/api/admin/pi/671aa75643075f8841becb27' \
--header 'api-key: your_api_key'
If you face any issues - Feel free to reach out to us at support@mockpi.co for more help!